views.pyを利用してデータベースにアクセスしてレコードを表示させます。
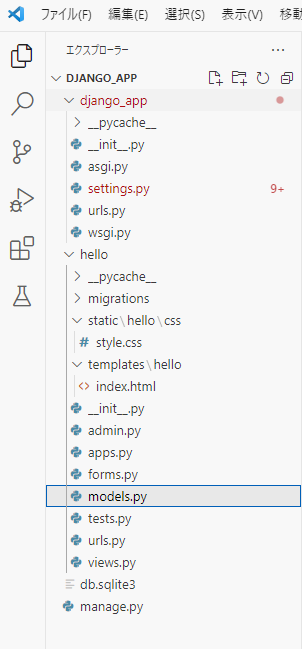
Contents
レコードの内容を表示する
hello/views.py
from django.shortcuts import render
from .models import Contact
def index(request):
data = Contact.objects.all()
params = {
'title': 'Hello',
'message': 'all contact.',
'data': data,
}
return render(request, 'hello/index.html', params)
indexではContactテーブルのデータをすべて取り出すのに下記のコードにて行っています。
data = Contact.objects.all()
モデルの内容を表示する
allで取り出したContactモデルのセットをテンプレートでテーブルにまとめて表示させます。
index,htmlを下記のように修正します。
hello/templates/index.html
<!doctype html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>hello</title>
</head>
<body class="container">
<h1 class="display-4 text-primary">{{title}}</h1>
<p class="h5 mt-4">{{message|safe}}</p>
<table class="table">
<tr>
<th>ID</th>
<th>NAME</th>
<th>GENDER</th>
<th>MAIL</th>
<th>AGE</th>
<th>BIRTHDAY</th>
</tr>
{% for item in data %}
<tr>
<td>{{item.id}}</td>
<td>{{item.name}}</td>
<td>{% if item.gender == False %}male{% endif %}
{% if item.gender == True %}female{% endif %}</td>
<td>{{item.mail}}</td>
<td>{{item.age}}</td>
<td>{{item.birthday}}</td>
<tr>
{% endfor %}
</table>
</body>
</html>
hello/urls.py
from django.urls import path
from . import views
urlpatterns = [
path('', views.index, name='index'),
]
webブラウザにてアクセスします。
Contactテーブルの内容が表示されていることを確認します。
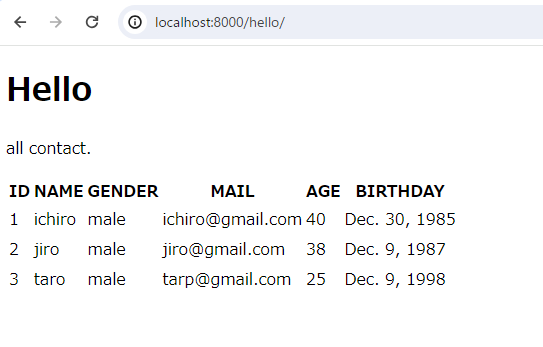
指定のIDのレコードだけ取り出す
指定のIDのレコードを取り出すにはフォームを用意する必要があります。
forms.pyを下記のように修正します。
hello/forms.py
from django import forms
class HelloForm(forms.Form):
id = forms.IntegerField(label='ID')
index.htmlを修正します。
hello/templates/index.html
<!doctype html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>hello</title>
</head>
<body class="container">
<h1 class="display-4 text-primary">{{title}}</h1>
<p class="h5 mt-4">{{message|safe}}</p>
<form action="{% url 'index' %}" method="post">
{% csrf_token %}
{{ form }}
<input type="submit" value="click">
</form>
<hr>
<table class="table">
<tr>
<th>ID</th>
<th>NAME</th>
<th>GENDER</th>
<th>MAIL</th>
<th>AGE</th>
<th>BIRTHDAY</th>
</tr>
{% for item in data %}
<tr>
<td>{{item.id}}</td>
<td>{{item.name}}</td>
<td>{% if item.gender == False %}male{% endif %}
{% if item.gender == True %}female{% endif %}</td>
<td>{{item.mail}}</td>
<td>{{item.age}}</td>
<td>{{item.birthday}}</td>
<tr>
{% endfor %}
</table>
</body>
</html>
ビュー関数を下記のように修正します。
hello/views.py
from django.shortcuts import render
from .models import Contact
from .forms import HelloForm
def index(request):
params = {
'title': 'Hello',
'message': 'all friends.',
'form':HelloForm(),
'data': [],
}
if (request.method == 'POST'):
num=request.POST['id']
item = Contact.objects.get(id=num)
params['data'] = [item]
params['form'] = HelloForm(request.POST)
else:
params['data'] = Contact.objects.all()
return render(request, 'hello/index.html', params)
webブラウザにてアクセスします。
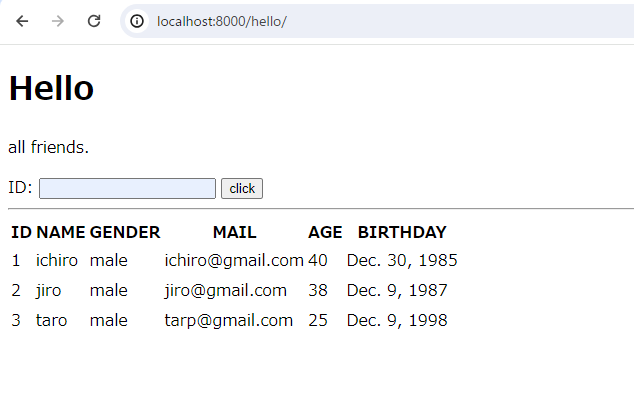
IDを指定して「click」をクリックします。
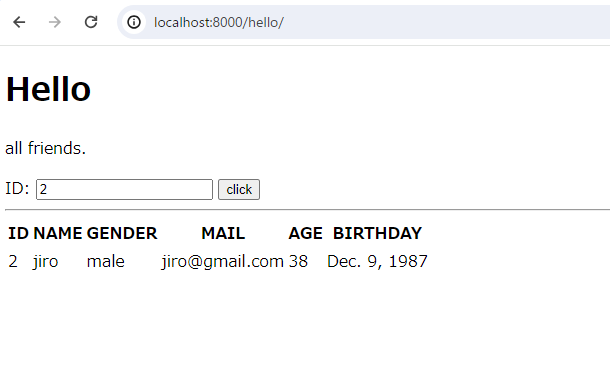
Managerクラスとは
ここまで、Contact.objectのallやgetといったメソッドを使って、レコードをContactインスタントとして取り出してきました。このContact.objectは「Manager」というクラスのインスタンスが入っています。
「Manager」はデータベースクエリを操作するためのクラスとなります。
モデルのリストを調べてみる
取り出したレコードのオブジェクトについて調べてみましょう。
views.pyを下記のように修正します。
hello/views.py
from django.shortcuts import render
from .models import Contact
def index(request):
data = Contact.objects.all()
params = {
'title': 'Hello',
'data': data,
}
return render(request, 'hello/index.html', params)
index.htmlを下記のように修正します。
hello/templates/index.html
<!doctype html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>hello</title>
</head>
<body class="container">
<h1 class="display-4 text-primary">{{title}}</h1>
<p class="h6 mt-4">{{data}}</p>
<table class="table">
<tr>
<th>data</th>
</tr>
{% for item in data %}
<tr>
<td>{{item}}</td>
<tr>
{% endfor %}
</table>
</body>
</html>
webブラウザにてアクセスします。
取り出したオブジェクトをそのままテキストで表示させています。
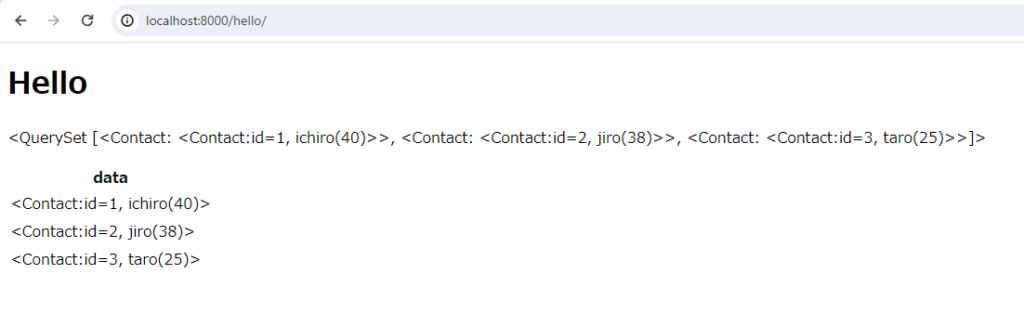
allで得られるのは「QuerySet」
valuesメソッドについて
レコードの値だけ欲しい場合は、valuesメソッドを利用します。
views.pyのindex関数を下記のように修正します。
hello/views.py
from django.shortcuts import render
from .models import Contact
def index(request):
data = Contact.objects.all().values()
params = {
'title': 'Hello',
'data': data,
}
return render(request, 'hello/index.html', params)
index.htmlから下記部分を削除しておきます。
<p class="h6 mt-4">{{data}}</p>
hello/templates/index.html
<!doctype html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>hello</title>
</head>
<body class="container">
<h1 class="display-4 text-primary">{{title}}</h1>
<table class="table">
<tr>
<th>data</th>
</tr>
{% for item in data %}
<tr>
<td>{{item}}</td>
<tr>
{% endfor %}
</table>
</body>
</html>
webブラウザにてアクセスします。
アクセスするとContactの内容が辞書の形で表示されます。
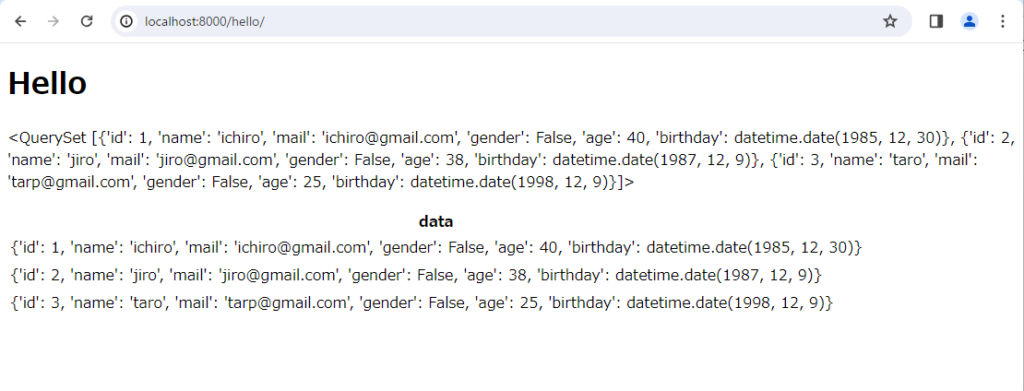
valuesメソッドにて特定の項目だけ取り出す
valuesメソッドでは引数に項目名を書いておくと、その項目の値だけ取り出すことができます。
views.pyのindex関数を下記のように修正します。
hello/views.py
from django.shortcuts import render
from .models import Contact
def index(request):
data = Contact.objects.all().values('id', 'name')
params = {
'title': 'Hello',
'data': data,
}
return render(request, 'hello/index.html', params)
webブラウザにてアクセスします。
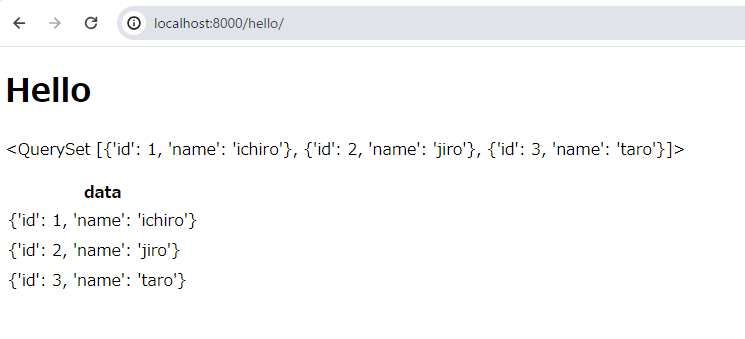
リストとして取り出す
QuerySetには取り出したモデルをリストとして取り出すメソッドもあります。
これは「value_list」というもので使い方はvaluesと同じです。
views.pyのindex関数を下記のように修正します。
hello/views.py
from django.shortcuts import render
from .models import Contact
def index(request):
data = Contact.objects.all().values_list('id','name','age')
params = {
'title': 'Hello',
'data': data,
}
return render(request, 'hello/index.html', params)
webブラウザにてアクセスします。
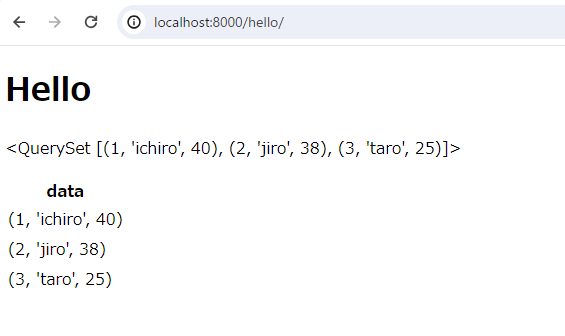
リストから最初と最後、レコード数を取得する
レコードの取得には他に下記のようなメソッドが用意されています。
first | allで取得したレコードの内、最初のものだけを返すメソッド |
last | allで取得したレコードの内、最後のものだけを返すメソッド |
count | 取得したレコード数を返すメソッド |
views.pyのindex関数を下記のように修正します。
hello/views.py
from django.shortcuts import render
from .models import Contact
def index(request):
num = Contact.objects.all().count()
first = Contact.objects.all().first()
last = Contact.objects.all().last()
data = [num, first, last]
params = {
'title': 'Hello',
'data': data,
}
return render(request, 'hello/index.html', params)
webブラウザにてアクセスします。
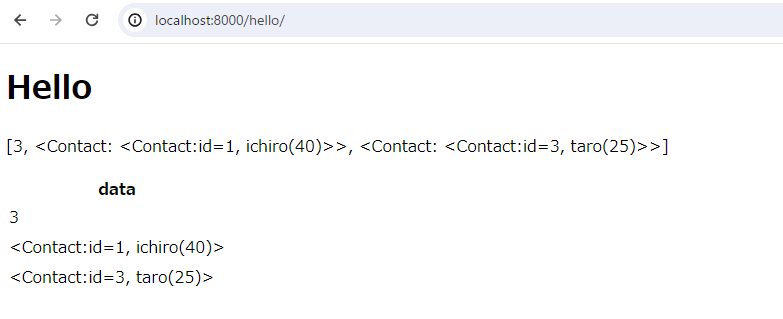
QuerySetの表示をカスタマイズ
データを見やすくカスタマイズします。
views.pyを下記のように修正します。
hello/views.py
from django.shortcuts import render
from .models import Contact
from django.db.models import QuerySet
def __new_str__(self):
result = ''
for item in self:
result += '<tr>'
for k in item:
result += '<td>' + str(k) + '=' + str(item[k]) + '</td>'
result += '</tr>'
return result
QuerySet.__str__ = __new_str__
def index(request):
data = Contact.objects.all().values('id', 'name', 'age')
params = {
'title': 'Hello',
'data': data,
}
return render(request, 'hello/index.html', params)
index.htmlを下記のように修正します。
hello/templates/index.html
<!doctype html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>hello</title>
</head>
<body class="container">
<h1 class="display-4 text-primary">{{title}}</h1>
<table class="table">
{{data|safe}}
</table>
</body>
</html>
webブラウザにてアクセスします。
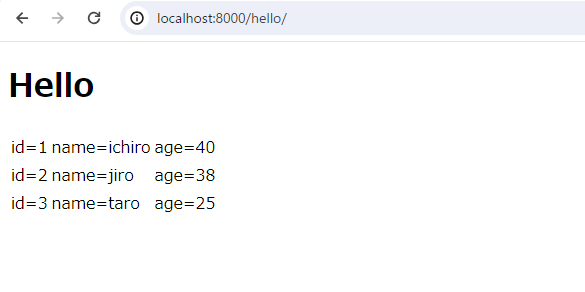
この記事は役に立ちましたか?
もし参考になりましたら、下記のボタンで教えてください。
コメント