複数の項目から1つを選ぶラジオボタンを作成します。
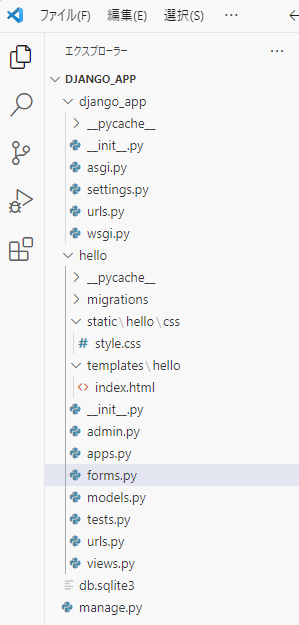
hello/views.py
from django.shortcuts import render
from django.http import HttpResponse
from django.views.generic import TemplateView
from .forms import HelloForm
class HelloView(TemplateView):
def __init__(self):
self.params = {
'title': 'Hello',
'form': HelloForm(),
'result':None
}
def get(self, request):
return render(request, 'hello/index.html', self.params)
def post(self, request):
ch = request.POST['choice']
self.params['result'] = 'selected: "' + ch + '".'
self.params['form'] = HelloForm(request.POST)
return render(request, 'hello/index.html', self.params)
hello/urls.py
from django.urls import path
from .views import HelloView
urlpatterns = [
path('', HelloView.as_view(), name='index'),
]
hello/templates/index.html
<!doctype html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>hello</title>
</head>
<body class="container">
<h1 class="display-4 text-primary">{{title}}</h1>
<p class="h5 mt-4">{{result|safe}}</p>
<form action="{% url 'index' %}" method="post">
{% csrf_token %}
<table>
{{ form.as_p }}
<tr><td></td><td>
<input type="submit" class="btn btn-primary my-2"
value="click">
</table>
</form>
</body>
</html>
forms.pyにチェックボックス関数を定義します。
hello/forms.py
from django import forms
class HelloForm(forms.Form):
data=[
('one', 'radio 1'),
('two', 'radio 2'),
('three', 'radio 3')
]
choice = forms.ChoiceField(label='radio', \
choices=data, widget=forms.RadioSelect())
webブラウザにてアクセスします。
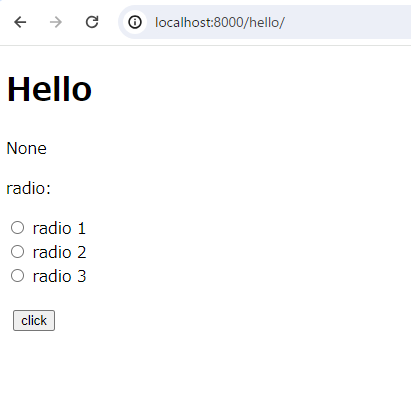
ラジオボタンから一つを選択して「click」をクリックします。
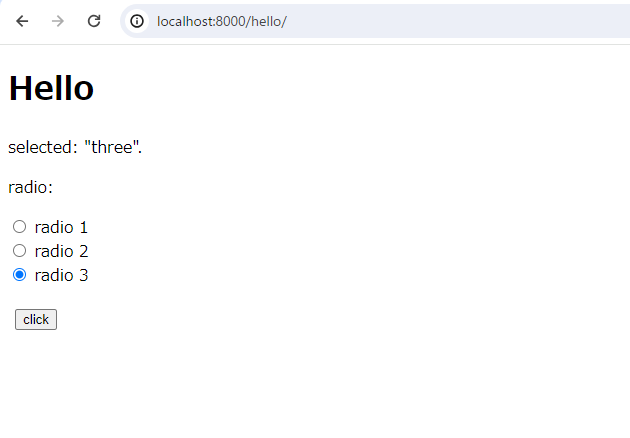
この記事は役に立ちましたか?
もし参考になりましたら、下記のボタンで教えてください。
コメント