データ一覧画面から、データを選択して削除を行います。
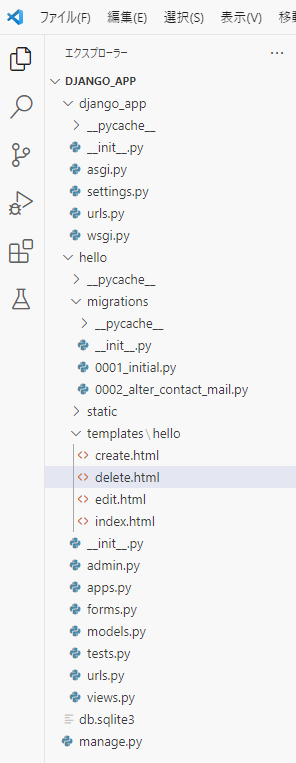
まずはdelete.htmlを作成します。
hello/templates/hello/delete.html
{% load static %}
<!doctype html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>{{title}}</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap/dist/css/bootstrap.css"
rel="stylesheet" crossorigin="anonymous">
</head>
<body class="container">
<h1 class="display-4 text-primary">
{{title}}</h1>
<p>※以下のレコードを削除します。</p>
<table class="table">
<tr><th>ID</th><td>{{obj.id}}</td></tr>
<tr><th>Name</th><td>{{obj.name}}</td></tr>
<tr><th>Gender</th><td>
{% if obj.gender == False %}male{% endif %}
{% if obj.gender == True %}female{% endif %}</td></tr>
<tr><th>Email</th><td>{{obj.mail}}</td></tr>
<tr><th>Age</th><td>{{obj.age}}</td></tr>
<tr><th>Birth</th><td>{{obj.birthday}}</td></tr>
<form action="{% url 'delete' id %}" method="post">
{% csrf_token %}
<tr><th></th><td>
<input type="submit" value="click"
class="btn btn-primary">
</td></tr>
</form>
</table>
</body>
</html>
views.pyにdelete関数を作成します。
hello/views.py
from django.shortcuts import render
from django.shortcuts import redirect
from .models import Contact
# from .forms import HelloForm #この文を削除する
from .forms import ContactForm #この文を新たに追記
def index(request):
data = Contact.objects.all()
params = {
'title': 'Hello',
'data': data,
}
return render(request, 'hello/index.html', params)
# create model
def create(request):
if (request.method == 'POST'):
obj = Contact()
contact = ContactForm(request.POST, instance=obj)
contact.save()
return redirect(to='/hello')
params = {
'title': 'Hello',
'form': ContactForm(),
}
return render(request, 'hello/create.html', params)
def edit(request, num):
obj = Contact.objects.get(id=num)
if (request.method == 'POST'):
contact = ContactForm(request.POST, instance=obj)
contact.save()
return redirect(to='/hello')
params = {
'title': 'Hello',
'id':num,
'form': ContactForm(instance=obj),
}
return render(request, 'hello/edit.html', params)
def delete(request, num):
contact = Contact.objects.get(id=num)
if (request.method == 'POST'):
contact.delete()
return redirect(to='/hello')
params = {
'title': 'Hello',
'id':num,
'obj': contact,
}
return render(request, 'hello/delete.html', params)
urls.pyにurlpatternsを追記します。
hello/urls.py
from django.urls import path
from . import views
urlpatterns = [
path('', views.index, name='index'),
path('create', views.create, name='create'),
path('edit/<int:num>', views.edit, name='edit'),
path('delete/<int:num>', views.delete, name='delete'),
]
データ一覧画面から削除できるように、index.htmlも修正します。
hello/templates/hello/index.html
<!doctype html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>hello</title>
</head>
<body class="container">
<h1 class="display-4 text-primary">
{{title}}</h1>
<table class="table">
<tr>
<th>data</th><th></th><th></th>
</tr>
{% for item in data %}
<tr>
<td>{{item}}</td>
<td><a href="{% url 'edit' item.id %}">Edit</a></td>
<td><a href="{% url 'delete' item.id %}">Delete</a></td>
<tr>
{% endfor %}
</table>
</body>
</html>
webブラウザにてアクセスします。
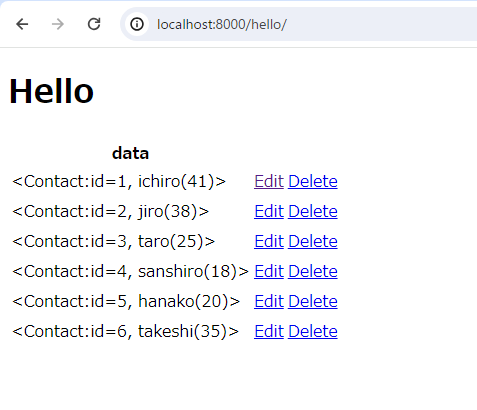
id=6のDeleteリンクをクリックします。
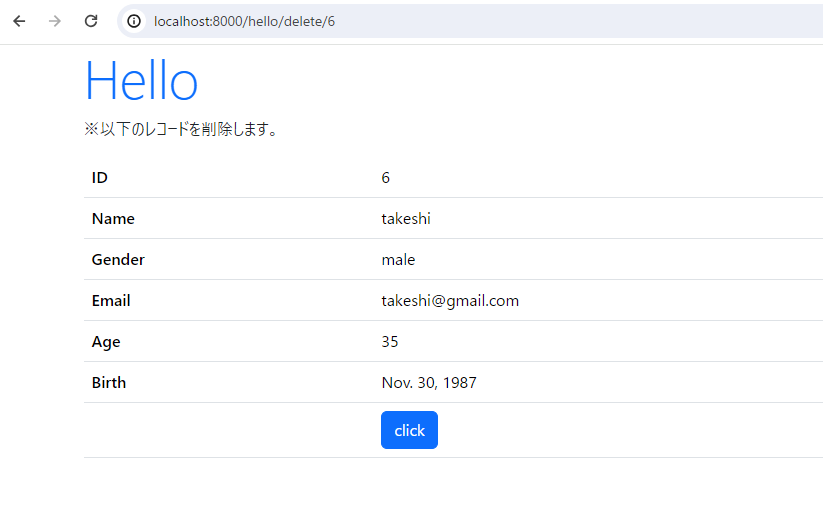
ID=6のデータは削除されました。
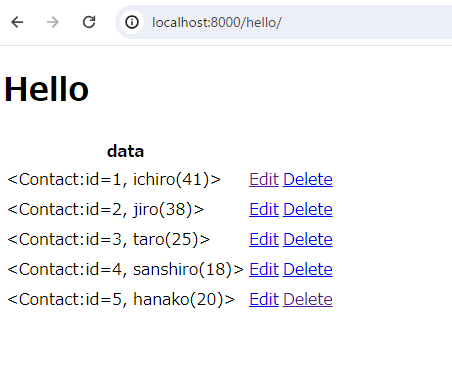
この記事は役に立ちましたか?
もし参考になりましたら、下記のボタンで教えてください。
コメント